10. April 2020
Rapid Prototyping with CSS Grid Layout
How to Create Easy and Fast Flexible Website Prototypes
In the following article I would like to show you how you can create useful and presentable website prototypes without in-depth knowledge of all specifications of the CSS Grid Layout Module. The result is a basic template for website prototypes that is flexible and easy to extend.
If it must go fast ...
Sometimes it just has to go fast. Be it because a customer is impatient, be it because we want to design our own website and have little time. Or it pushes us again to get started without a big plan.
Then the normal way from idea to finished product is too far: conception and planning, content creation, drawing or Sketch, clickdummy, PhotoShop or InVision, more prototypes, 1st draft, 2nd draft ..., and finally the launch (publication) of the website.
And frameworks like Bootstrap, Foundation etc. are also out of the question, because the effort is too big or the learning curve is too high.
... Rapid Prototyping is the means of choice
Here the method Rapid Prototyping is useful. This can be implemented effectively with CSS Grid Layout.
With the approach of Rapid Protyping the whole process of website development can be done directly and almost exclusively in a browser.
Advantages of Rapid Prototyping
- All functionalities of CSS (animations, hover effects etc.) can be used
- Presentation to clients in early developmental stage possible
- Errors can be quickly detected and fixed
- Testing of functionality and design immediately in the browser
- Time and costs are saved ("resource-saving")
What You Should Bring with You
Knowledge of HTML and CSS is required, knowledge of CSS Grid Layout is desirable. At the end of this article you will find a link list with further information about CSS Grid.
For those in a Hurry
See the Demo Site for easier comprehension.
You don't have to read the whole article step by step, you can also view the source code on CodePen and download it there or "fork" it, i.e. create your own version from my template. How this works you can learn here and here.
The Result
The following graphic shows the final result of the website prototype.
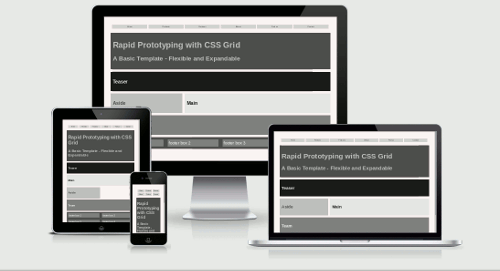
The Result: A Template for Rapid Prototyping with CSS Grid Layout
This is only one of many possible variants.
The Markup
The basic HTML structure is created quickly and easily. A navigation, a header, a teaser, the element main for the main content and the element aside for the sub-content as well as a footer are inserted into the grid container. Finally, four boxes are added to the footer as Sub Grids.
HTML-Code <div class="grid-container"> <nav> <ul class="grid-nav-main"> <li><a href="">Home</a></li> <li><a href="">Products</a></li> <li><a href="">Features</a></li> <li><a href="">About</a></li> <li><a href="">Find us</a></li> <li><a href="">Contact</a></li> </ul> </nav> <header class="grid-box"> <h1>Header</h1> <h2>Rapid Prototyping mit CSS Grid</h2> </header> <div class="grid-box teaser"> <h3>Teaser</h3> </div> <main class="grid-box"> <h3>Main</h3> </main> <aside class="grid-box"> <h3>Aside</h3> </aside> <footer class="grid-box sub-grid"> <div class="sub-grid-box"> footer box 1 </div> <div class="sub-grid-box"> footer box 2 </div> <div class="sub-grid-box"> footer box 3 </div> <div class="sub-grid-box"> footer box 4 </div> </footer> </div>
Advantages of CSS Grid Layout
With the module CSS-Grid-Layout there is finally a technique with which you can build responsive and very flexible websites. With the grid any number of columns and rows can be created and within this grid contents can be arranged horizontally and vertically freely.
Grid Layout allows a consistent separation of content and design. HTML elements can be structured and designed via CSS without affecting the HTML markup.
However, you should be very sparing in changing the display of the HTML code with CSS, because now the HTML structure differs from the display of the content on the screen. This is no longer accessible, since people with visual impairments, for example, use screenreaders, which only read out the content of the HTML document in linear sequence. Changes via CSS are not taken into account.
Design with CSS Grid Areas
With Grid Areas you can precisely name and position the areas that should appear on the frontend.
Examples:
- The navigation fills the area "nav" (grid-area: nav)
- The footer is given the grid-area name "footer" (grid-area: footer)
Advantage: The name of the area matches the function. Of course you are completely free in the naming of the grid-areas. The names only have to match in HTML and CSS code.
Defining the Grid Areas
In the first step I define the following Grid Areas:
- Navigation
- Header
- Teaser
- Main
- Aside
- Team (will be added later) and
- Footer
The appropriate code:
CSS-Code /* Defining the grid areas */ nav { grid-area: nav; } header { grid-area: header; } .teaser { grid-area: teaser; } main { grid-area: main; } aside { grid-area: aside; } footer { grid-area: footer; }
On screens up to 500 pixels in size, the areas in a column should appear one below the other.
On screens that are between 500 and 800 pixels in size, the areas are displayed in two columns. The area aside gets only one column.
On screens larger than 800 pixels, the areas are arranged in three columns, where aside (1 column) is to be to the left of main (2 columns).
The Grid Layout - display: grid
I place the grid layout in the container with the class "grid-container". With the CSS statement "display: grid" the div element becomes a container for the Grid Layout – that's all it needs.
CSS-Code /* Styles for the grid container */ .grid-container { display: grid; grid-gap: 1rem; }
With grid-gap I specify the distance between the grid areas; here I choose 1rem, which usually corresponds to 16 pixels.
In the future the CSS property grid-gap will be replaced by gap. Until all browsers have implemented this, you can stay with grid-gap or write as fallback "grid-gap: 1rem;" and below "gap: 1rem;".
The Single Grid Areas
In the next step I specify the grid areas and where they should appear on the screen. Here I stick to the structure of the HTML document for now.
CSS-Code /* Styles for the grid container */ .grid-container { display: grid; /* Styles for the placement of the grid areas */ grid-template-areas: "nav" "header" "teaser" "main" "aside" "footer"; grid-gap: 1rem; }
With this CSS code, the HTML elements are displayed one below the other on all screen sizes. To change this, in the next step I use Media Queries.
The Single Grid Areas on Different Screen Sizes
I will insert two media queries into the CSS code to explain the principle. For prototypes these two media queries may be sufficient, for a finished website several media queries may be necessary.
Media Query for Screens larger than 500 Pixels
First I determine how the display should look for screens larger than 500 pixels and smaller than 800 pixels. Again, I leave the order as it was defined in the HTML code. All grid areas should be two columns wide, but the area aside should only be one column wide. An empty column can be marked by a dot:
CSS-Code /* Media queries for the grid container > 500px */ @media only screen and (min-width: 500px) { .grid-container { grid-template-columns: repeat(2,1fr); grid-template-areas: "nav nav" "header header" "teaser teaser" "main main" "aside ." "footer footer"; } }
With grid-template-columns: repeat(2,1fr); I instruct the browser to display the content in two ("repeat "+"2") equally wide ("1fr") columns ("grid-template-columns"). The unit "fr" means "fraction". Here 1fr means that each column gets an equal share of the free space, i.e. 50% of the space left by the screen or a surrounding container.
Media Query for Screens larger than 800 Pixels
The same is true for the instructions for screens larger than 800 pixels, except that I now split the content into three columns ("repeat(3,1fr)"). The container aside (1 column) now comes to the left of the container main (2 columns).
CSS-Code /* Media queries for the grid container > 800px */ @media only screen and (min-width: 800px) { .grid-container { grid-template-columns: repeat(3,1fr); grid-template-areas: "nav nav nav" "header header header" "teaser teaser teaser" "aside main main" "footer footer footer"; } }
Create Variants with Grid Areas
The structure of the areas can be extended or shortened and redesigned at will.
Hierarchization of Content with Grid Areas
If, for example, the area main should appear on screens up to 500 pixels above teaser, this can easily be done with the following CSS statement. We simply exchange teaser with main in the grid container under grid-template-areas. You can arrange the grid areas according to your requirements – for any desired screen size. The example code is:
CSS-Code /* Styles for the grid container */ .grid-container { display: grid; /* Styles for the placement of the grid areas */ grid-template-areas: "nav" "header" "main" "teaser" "aside" "footer"; grid-gap: 1rem; }
Expansion of the Grid Areas
The new area team should be added to the existing grid areas under the area aside. Of course, you can define any area as a grid area and place it where it suits you. First I add a div-container with the class team to the HTML code:
HTML-Code ... <aside class="grid-box"> <h3>Aside</h3> </aside> <div class="grid-box team"> <h3>Team</h3> </div> ...
Now I extend the definition of the grid areas by the area team:
CSS-Code /* Add 'Team' the grid areas definition */ ... .team { grid-area: team; } ...
In the next step I insert the area team into the grid container in the CSS code under "grid-template-areas" where I want to see it on the screen – in this case under aside:
CSS-Code .grid-container { display: grid; grid-template-areas: "nav" "header" "teaser" "main" "aside" "team" "footer"; grid-gap: 1rem; }
I extend the CSS code in both Media Queries accordingly.
Finally I style the div container team with a little bit CSS:
CSS-Code .team { background-color: #7F7F7F; color: #F2F2F2; }
Nested Grids
Within a grid, you can create additional grids as subgrids. I define them as described above for the grid container.
For subgrids an official specification is in progress, but not yet adopted. Meanwhile I use the following code, which works well. First the HTML code:
HTML-Code <footer class="grid-box sub-grid"> <div class="sub-grid-box footer-box-1"> footer box 1 </div> <div class="sub-grid-box footer-box-2"> footer box 2 </div> <div class="sub-grid-box footer-box-3"> footer box 3 </div> <div class="sub-grid-box footer-box-4"> footer box 4 </div> </footer>
The footer receives the class sub-grid and becomes the grid container of the four child elements with the class sub-grid-box through "display: grid". Then I style the subgrid boxes with three lines of CSS.
I proceed here again as in the above example for the grid container. On screens up to 800 pixels in size, the subgrids are displayed in two columns and two rows, from 800 pixels then in one row and in four columns.
And here's the matching CSS code:
CSS-Code /* Defining the subgrid areas */ .footer-box-1 { grid-area: footer-box-1; } .footer-box-2 { grid-area: footer-box-2; } .footer-box-3 { grid-area: footer-box-3; } .footer-box-4 { grid-area: footer-box-4; } /* Styles for the nested grid container */ .sub-grid { display: grid; grid-template-columns: repeat(2,1fr); grid-template-areas: "footer-box-1 footer-box-2" "footer-box-3 footer-box-4"; grid-gap: 1rem; } /* Media query for the nested grid container */ @media only screen and (min-width: 800px) { .sub-grid { grid-template-columns: repeat(4,1fr); grid-template-areas: "footer-box-1 footer-box-2 footer-box-3 footer-box-4"; } } /* Styles for the sub-grid items */ .sub-grid-box { padding: .5rem; background-color: #7F7F7F; color: #fff; }
Create an easy Navigation with CSS Grid
Finally I create a navigation, which should be sufficient in most cases for a prototype.
As usual, I create a list that contains the navigation elements. The list ul (HTML element "unordered list") becomes a grid container and the list contents li (HTML element "list item") become grid items.
HTML-Code <nav> <ul class="grid-nav-main"> <li><a href="">Home</a></li> <li><a href="">Products</a></li> <li><a href="">Features</a></li> <li><a href="">About</a></li> <li><a href="">Find us</a></li> <li><a href="">Contact</a></li> </ul> </nav>
With Media Queries I adjust the navigation to different screen sizes. On smaller screens, three navigation elements are displayed side by side in three columns and two rows; on larger screens, all six navigation elements are displayed in six columns and one row side by side.
Here is the CSS code for the navigaton:
/* Styles for the grid navigation - just for presentation or show - not for produce */ .grid-nav-main { display: grid; grid-template-columns: repeat(3,minmax(75px,1fr)); /* 3 links in a row */ grid-gap: .25rem; justify-content: center; align-items: center; margin: 0; padding: .5rem 0; list-style: none; } /* 2 media queries for the grid navigation */ @media only screen and (min-width: 380px) { .grid-nav-main { grid-template-columns: repeat(3,minmax(100px,1fr)); /* 3 links in a row */ } } @media only screen and (min-width: 550px) { .grid-nav-main { grid-template-columns: repeat(auto-fit,minmax(75px,1fr)); /* All links in one row */ } } /* Styles for the grid navigation links */ .grid-nav-main a { display: block; background: #D3D3D3; color: #333; font-size: .8rem; line-height: 1; margin: 0; padding: .5rem 0; text-align: center; text-decoration: none; transition: all .75s ease; } .grid-nav-main a:hover { background-color: #333; color: #D3D3D3; } /* Media query for the grid navigation links */ @media only screen and (min-device-width: 800px) { .grid-nav-main a { font-size: 1rem; line-height: 1.2; padding: 1rem; text-align: center; } }
Explanations
Gives the summed width of the navigation elements more than the width of the Viewport, the remaining elements are wrapped and arranged in a second row. Thus the navigation is already responsive without media queries.
For screens smaller than 550 pixels
In order to prevent a single navigation element from being wrapped into a second row on narrower screens, for example, I use grid-template-columns: repeat(3,minmax(75px,1fr)) and grid-template-columns: repeat(3,minmax(100px,1fr)) to instruct smaller screens to display three elements in a row. This results in two lines for six navigation elements.
For screens larger than 550 pixels
The CSS code grid-template-columns: repeat(auto-fit,minmax(75px,1fr)); means that the navigation elements are at least 75px wide; the maximum size is defined by "1fr": The available space of the Viewport is split evenly ("auto-fit") between the navigation elements.
Excursus: Calculation of the Minimum Width of the Viewport
I have set a media query with min-width 550px. This means that the CSS information becomes effective when the Viewport is at least 550 pixels wide. The navigation has six elements that are to be displayed next to each other from this width on.
This is based on the following calculation:
- 6 columns with the minimum width of 75px: 6x75px = 450px
- Gap between the columns (grid-gap: .25rem=4px, because 1rem = 16px): 5x4px = 20px
- Margin of body: 2x2rem = 2x32px = 64px
- Padding of the grid container: 2x.5rem = 2x8px = 16px
- Gives the sum: 550px = minimum width of the Viewport
It is easier than this calculation to try it out with trial and error and place the media queries where they fit. This works well in a browser with developer tools or on various suitable devices running browsers that support CSS Grid Layout.
Click here to go to the Demo Site.
You can also view the source code on CodePen and download it there or "fork" it, i.e. create your own version from my template. How this works you can learn here and here.
Links
Short introductions to the CSS Grid Layout Module are available here and here.
If you want more: quakit.com has a Tutorial to CSS Grid Layout.
A useful helper to keep track of is the cheat sheet at malven.co.
CSS Grid Layout can be learned playfully in the Grid Garden.
A wonderful overview of the possibilities to design websites with CSS Grid offers Rachel Andrew.
Detailed guides or overviews can be found on the following websites: css-tricks.com and tympanus.net.
You can find a "reference book" at Mozilla Development Network.
A German introduction is available at the Kulturbanause.
On the state of affairs (July 2018) regarding subgrids, please read the article in the Smashingmagazine.
More information about Rapid Prototyping is available here and here.