24. June 2020
How to Create an Image Gallery with CSS Grid Layout
Responsive without Media Queries
Whether you want to design a picture gallery, a portfolio or a card layout, you are always faced with the problem of how this looks on different screen sizes. Media queries are usually used for this. But there is a solution that does not require media queries at all. I would like to show you how this works by means of a simple picture gallery.
The Basics
As a framework I use a grid, which is designed with CSS Grid Layout. The images are then placed in frames, for which I use CSS Flexbox.
The structure of the HTML code looks like this:
HTML Code
<div class="gallery-grid"> <figure class="gallery-frame"> <img class="gallery-img" src="https://picsum.photos/230/300?random=1" alt="Image form https://picsum.photos" title="Image form https://picsum.photos"> <figcaption>Image Title</figcaption> </figure> </div>
You can see the whole code on Codepen.
Excursus: Difference between auto-fill and auto-fit
In the CSS code for the image gallery I create the columns with the CSS declaration
grid-template-columns: repeat(auto-fill, minmax(200px,1fr));
or with
grid-template-columns: repeat(auto-fit, minmax(200px,1fr));
What makes the difference between both CSS values?
Suppose we have three boxes that should have a minimum width of 200 pixels. The maximum width of the parent container is 720 pixels wide.
With the CSS value auto-fill
the width of 720 pixels is divided into three columns and filled in: the three boxes fit into the existing width.
If we remove a box, the unused space remains free and visible with a total width of 720 pixels, so it is not filled in.
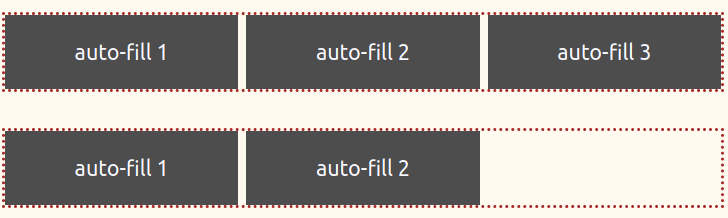
With the CSS value auto-fit
on the other hand, the entire width of a row is always filled in, regardless of how many columns are available. As long as all items fit into a row, the available space is distributed among the items. If the items take up more space than is available, the row is wrapped.
No matter if we take two or three boxes of 200 pixel width each, the 720px width of the container is always filled.
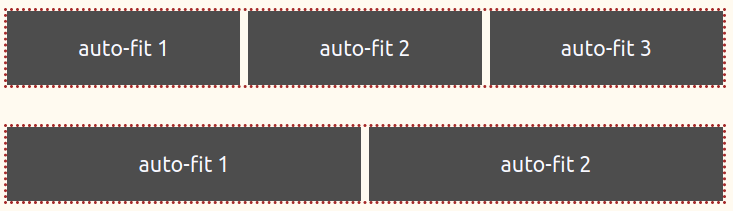
Notes
In both cases – auto-fill and auto-fit – the wrap is done automatically thanks to the following CSS declarations:grid-template-columns: repeat(auto-fill, minmax(200px,1fr));
and grid-template-columns: repeat(auto-fit, minmax(200px,1fr));
The columns should be filled repeatedly with HTML elements (paragraphs, images, articles, etc.) with a minimum width of 200 pixels and a maximum width of 1fr per element; 1fr (fraction unit) means an equal part of the available width. If the total width of all existing elements exceeds the available width, a wrap is automatically performed. This is done until only one HTML element fits into one line.
If we disregard margin, padding and border for the HTML elements, a wrap is made at 599 pixels (3*200px=600px) and another at 399 pixels (2*200px=400px).
The Image Gallery
The following picture gallery is designed according to the above scheme – I use here auto-fit
. Of course you can also display any other form of content in this way – cards, text blocks, product displays – or use it to design pages like portfolios, landing pages or catalogues.
First of all the CSS code for the image gallery:
CSS-Code
/* First the Grid */ .gallery-grid { display: grid; grid-template-columns: repeat(auto-fit, minmax(250px, 1fr)); grid-gap: 1.5rem; justify-items: center; margin: 0; padding: 0; } /* The Picture Frame */ .gallery-frame { padding: .5rem; font-size: 1.2rem; text-align: center; background-color: #333; color: #d9d9d9; } /* The Images */ .gallery-img { max-width: 100%; height: auto; object-fit: cover; transition: opacity 0.25s ease-in-out; } .gallery-img:hover { opacity: .7; }